前回、プレハブバリアントを使うことで、敵機の種類を増やすのが簡単になりました。今回は、調子に乗ってあといくつか敵機の種類を増やしてみたいと思います。
さらに敵機の種類を増やす
敵機の種類を増やす
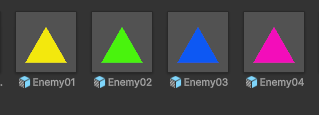
プレハブバリアントを使って、Enemy02からEnemy04までを作ってみました。色もそれぞれ変えています。あとは、これにスクリプトを付けていきます。
Enemy01
using UnityEngine;
public class Enemy01 : Enemy
{
public float roll = 0.5f; //反時計回りの角度0.5度
public int interval = 10;
// Start is called once before the first execution of Update after the MonoBehaviour is created
new void Start()
{
base.Start();
if (transform.position.x > 0) roll = -roll; //画面の右半分に生成されたときは、時計回りにする
}
// Update is called once per frame
new void Update()
{
base.Update();
if (lifeTime % interval == 0){
transform.Rotate(0f, 0f, roll);
}
rb.linearVelocity = transform.up * speed;
}
}
Enemy01は、中央より右側に出現した時は、時計回りに回転し、中央より左側に出現した時は、反時計回りに回転するようにしています。これにより、どちらの場合でも中央に向かっていくようにしました。
Update関数の処理内容を見てみます。base.Update()によって、継承元であるEnemyクラスのUpdate関数が呼び出され、lifeTimeが1つ増えたり、画面からはみ出したときに消滅したりします。その後、lifeTime % interval でわり算の余りをもとめ、これが0の時(lifeTimeがintervalで割り切れたとき=lifeTimeがintervalの倍数であるとき)指定された角度の回転をします。intervalが10であれば、10フレームに一度向きを変えることになります。ちなみに変数のlifeTimeはこのクラスでは宣言していませんが、継承元のクラスにある(継承元のクラスのメンバー)ので宣言なしで使えています。
Enemy02
using UnityEngine;
public class Enemy02 : Enemy
{
float f_timer = 0f;
// Start is called once before the first execution of Update after the MonoBehaviour is created
new void Start()
{
base.Start();
}
// Update is called once per frame
new void Update()
{
f_timer += 0.01f;
base.Update();
transform.Rotate(0f, 0f, Mathf.Sin(f_timer)/2);
rb.linearVelocity = transform.up * speed;
}
}
ゆらゆら左右に揺れる動きを表現したかったので、サイン(Sin)を使うことにします。Mathf.Sin(角度)の中で、角度を少しずつ増やしていく(f_timer += 0.01f)と、+1~-1の間で増えたり減ったりする値が得られるので、それをRotateの角度に入れてあげると、右に回転したり左に回転したりするようになります。そのままだと、回転角度が大きかったので、2で割って調整しています。
Enemy03
using UnityEngine;
public class Enemy03 : Enemy
{
// Start is called once before the first execution of Update after the MonoBehaviour is created
new void Start()
{
base.Start();
}
// Update is called once per frame
new void Update()
{
base.Update();
if (lifeTime >400 && lifeTime < 580)
{
transform.Rotate(0f, 0f, 1f);
}
rb.linearVelocity = transform.up * speed;
}
}
Enemy03はEnemy01と似ていますが、誕生して400フレーム後から、毎フレーム1度ずつ回転します。180フレーム経過すると回転を止めます。つまり180度回転して戻っていく動きになります。
Enemy04
using UnityEngine;
public class Enemy04 : Enemy
{
public float roll = 0.5f;
public int interval = 10;
// Start is called once before the first execution of Update after the MonoBehaviour is created
new void Start()
{
base.Start();
if (transform.position.x > 0) roll = -roll;
}
// Update is called once per frame
new void Update()
{
base.Update();
if (lifeTime % interval == 0){
transform.Rotate(0f, 0f, roll);
rb.linearVelocity = transform.up * speed;
}
if (lifeTime > 400 && lifeTime < 580)
{
transform.Rotate(0f, 0f, 1f);
}
}
}
Enemy04はEnemy01とEnemy03を合わせた動きをします。最初はEnemy01と同じように振る舞いますが、400フレーム経つと、反転していきます。
StageManager
敵機の増加に合わせてStageManagerも変更する必要があります。
using UnityEngine;
public class StageManager : MonoBehaviour
{
public GameObject Player;
public GameObject Enemy01;
public GameObject Enemy02;
public GameObject Enemy03;
public GameObject Enemy04;
int timer = 0;
const int timerMax = 400;
// Start is called once before the first execution of Update after the MonoBehaviour is created
void Start()
{
Instantiate(Player, new Vector3(0, -4.5f, 0), Quaternion.identity);
}
// Update is called once per frame
void Update()
{
timer++;
if (timer == 100) {
Instantiate(Enemy01, new Vector3(Random.Range(-10f, 10f), 6f, 0f), Quaternion.Euler(0, 0, 180));
} else if (timer == 200) {
Instantiate(Enemy02, new Vector3(-12f, 2f, 0f), Quaternion.Euler(0, 0, -140));
} else if (timer == 300) {
Instantiate(Enemy03, new Vector3(Random.Range(-10f, 10f), 6f, 0f), Quaternion.Euler(0, 0, 180));
} else if (timer == 400) {
Instantiate(Enemy04, new Vector3(Random.Range(-10f, 10f), 6f, 0f), Quaternion.Euler(0, 0, 180));
}
if (timer > timerMax) timer = 0;
}
}
まず、public GameObject Enemy01でEnemy01のプレハブを設定する場所を作ります。同じようにEnemy02からEnemy04までも作ります。※配列にしてもいいかもしれませんね。
次に、Update関数の方では、100フレームでEnemy01、200フレームでEnemy02というように、時間経過によって敵機を生成していきます。Enemy02だけは画面の左から右向きに進むように、発生場所と角度を調整していますが、その他は画面の上部からランダムに出現させています。400フレーム経過したら(全ての敵を出現させたら)またタイマーを0に戻して最初から出現させていきます。
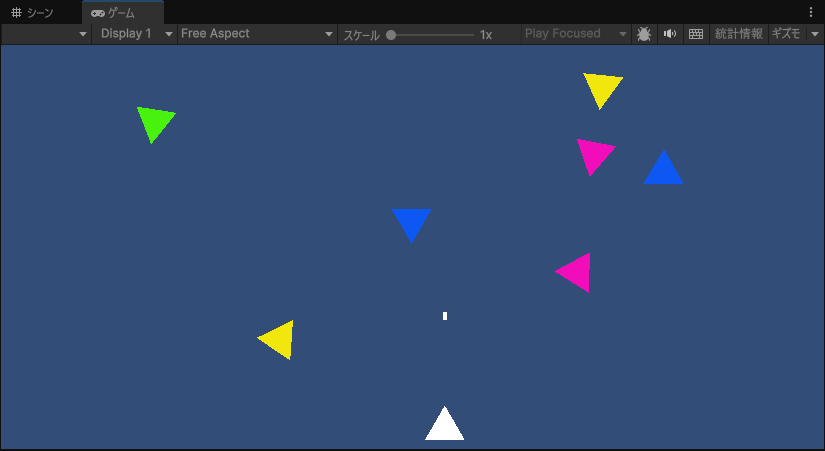
実行してみると、かなりにぎやかになってきました。
今回はここまでです。お疲れ様でした。
コメント